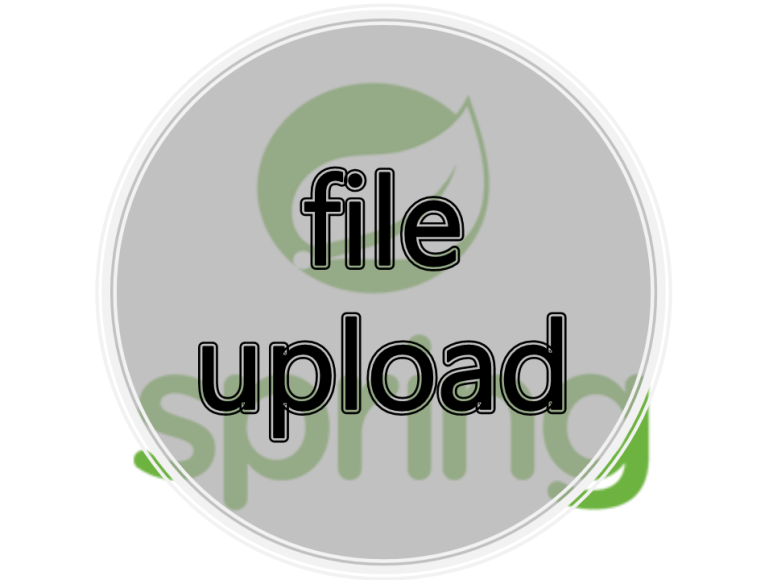
파일 업로드를 위한 프로젝트를 만들어 보겠습니다.
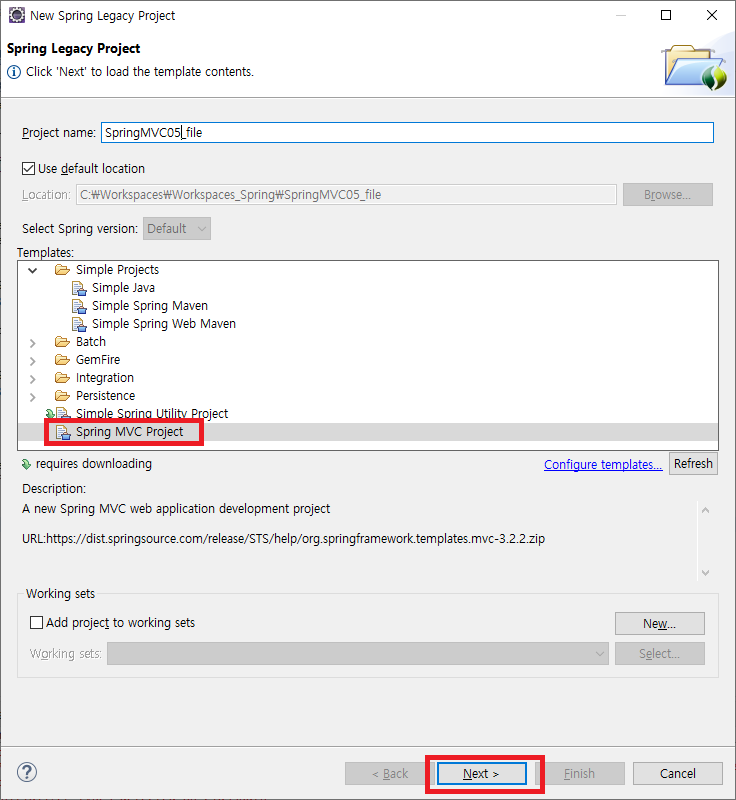
우선 legrcy 프로젝트를 만들어주세요!
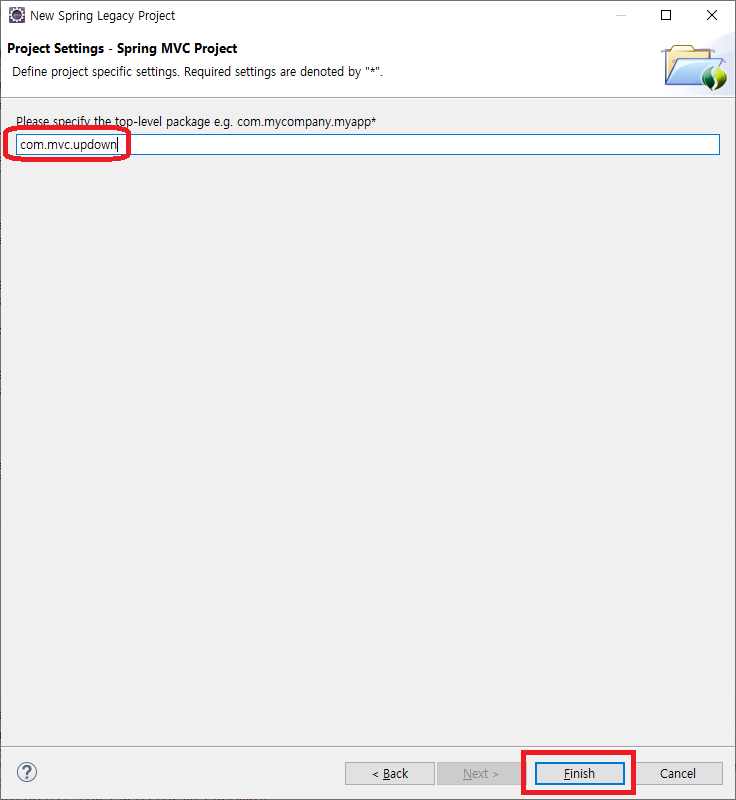
(저는 패키지 com.mvc.updown으로 만들겠습니다)
pom.xml에 디펜던시를 추가해줍니다!
commons-io.commons-io
commons-fileupload.commons-fileupload
io와 관련된것을 하기위해서 또 fileupload를 하기 위해 필요합니다!
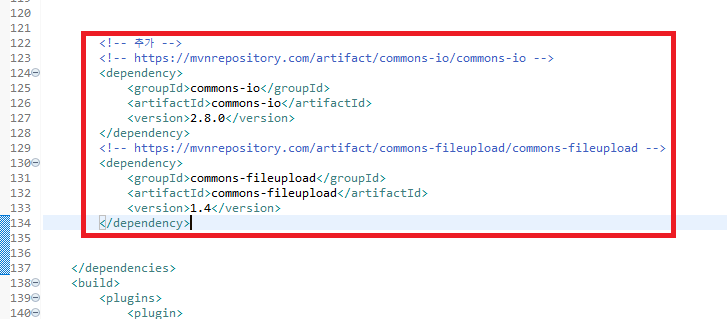
pom.xml
maven repository에는 잘 나오는데 search.org에는 없을수도 있다고 합니다!
web.xml로 이동합니다.
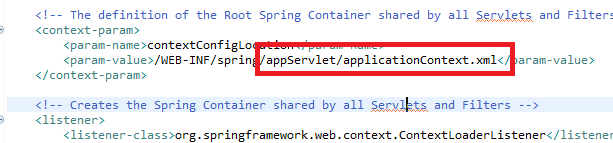
web.xml
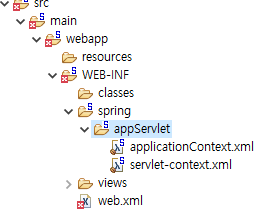
기존에 만들어져 있던 root-context.xml를 appServlet안으로 이동시키고 이름을 applicationContext.xml로 바꾸었습니다!(에러 이클립스 에러입니다 ㅠㅠ)
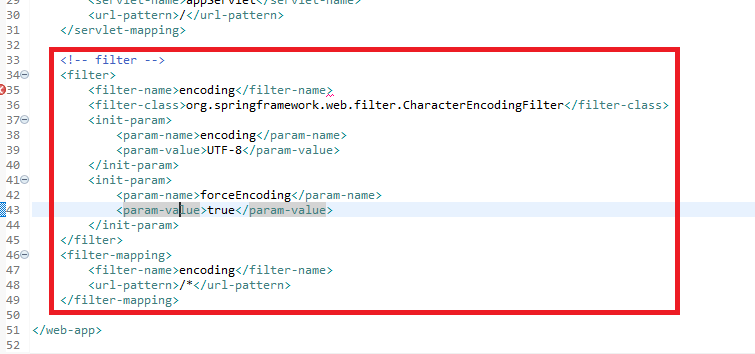
web.xml
그리고 아래쪽에 filter를 작성해주세요!
(filter-mapping 안해주면 실행시켰을때 글자 깨집니다!)

applicationContext.xml
그다음 applicationContext.xml에 다음과 같이 작성합니다.
multipartResolver가 멀티파트 파일을 받아줍니다.
최대 크기는 100000000로 지정해주었으며 UTF-8로 기본 인코딩 형태를 지정해주었네요.
maxUploadSize의 단위는 byte입니다.
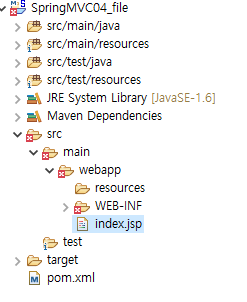
다음으로 webapp아래에 index.jsp파일을 만들겠습니다.(여기 에러 이클립스 에러입니다...!)
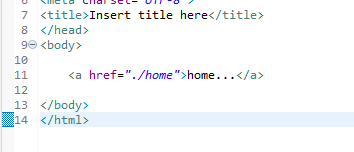
index.jsp
index에 다음과 같이 작성하겠습니다.
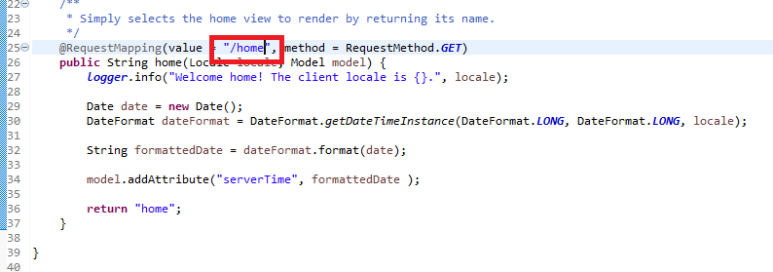
컨트롤러에서 /home으로 수정해줍니다.
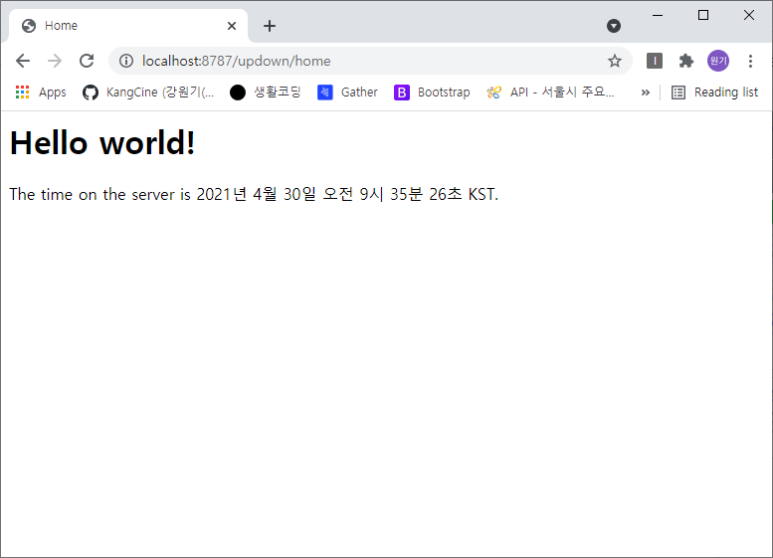
여기까지만 하면 이런 화면이 뜹니다!(경로이동 잘 되나 확인차 해보았습니다!)
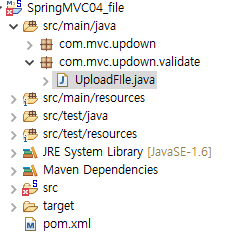
이제 com.mvc.updwon.validate패키지를 만들고 그 아래 UploadFile이라는 클래스를 만들겠습니다.
UplodaFile.java
package com.mvc.updown.validate;
import org.springframework.web.multipart.MultipartFile;
public class UploadFIle {
private String name;
private String desc;
private MultipartFile mpfile;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDesc() {
return desc;
}
public void setDesc(String desc) {
this.desc = desc;
}
public MultipartFile getMpfile() {
return mpfile;
}
public void setMpfile(MultipartFile mpfile) {
this.mpfile = mpfile;
}
}
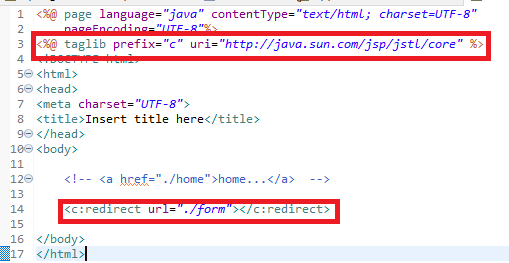
index.jsp
다시 index.jsp로 와서 a 주석처리 하고 taglib을 적고 다음과 같이 코드를 적어주세요!

HomeController.java
HomeController에 다음과 같은 코드로 작성해주세요!
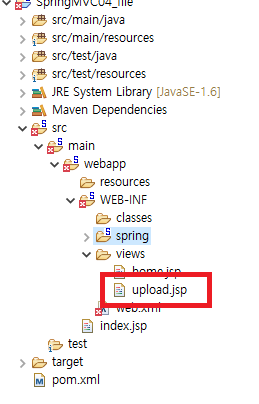
그러면 이제 upload.jsp를 만들어야겠죠?
upload.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="form" uri="http://www.springframework.org/tags/form" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<form:form method="post" enctype="multipart/form-data" modelAttribute="uploadFile" action="upload">
<h3>uploadForm</h3>
file<br/>
<input type="file" name="mpfile" /><br/>
<p style="color:red; font-weight: bold;">
<form:errors path="mpfile"></form:errors>
</p><br/>
<textarea rows="10" cols="40" name="desc"></textarea><br/>
<input type="submit" value="send" />
</form:form>
</body>
</html>
upload.jsp파일에 taglib을 form으로 잡아주시고 바디를 작성해주세요!
form태그 안에 enctype="multipart/form-date"로 잡혀있습니다
enctype속성의 값으로는
1. application/www-form-urlencoded : (default) 문자들을 encoding
2. multipart/form-date : file upload - post 방식만 가능
3. text/plain : encoding 하지 않음
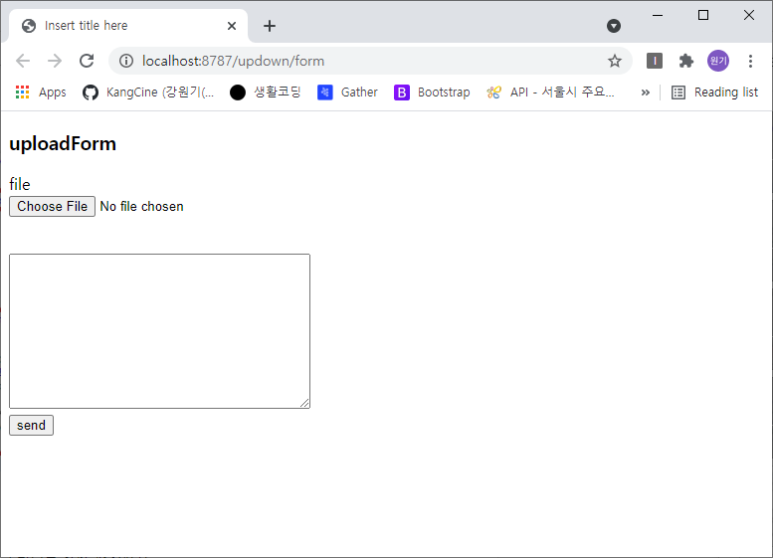
이제 다시 프로젝트를 실행 시키면 이런 화면이 나옵니다!
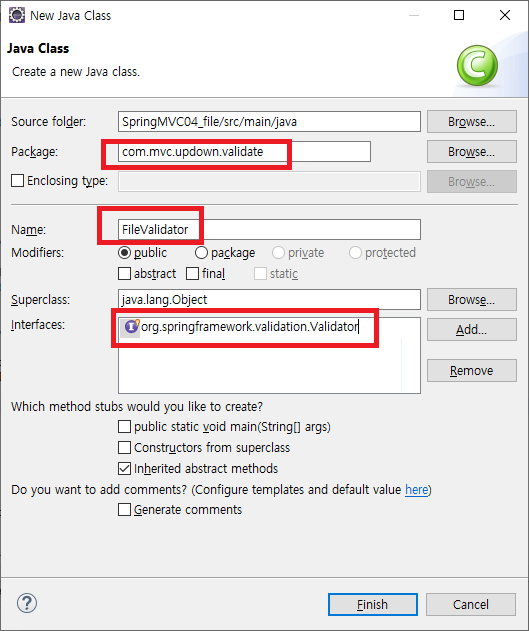
아까 만들어두었던 com.mvc.updown.validate 패키지 안에 FileValidator 클래스를 만들어줍니다!
만들떄 Validator 임포트 해주세요!
Validator는 유효성검사를 위해 사용합니다!
유효성 검사는프론트엔드(자바스크립트)에서도 할수 있지만 보안을 위해 백엔드에서 하는것이 안전합니다.
FileValidator.java
package com.mvc.updown.validate;
import org.springframework.stereotype.Service;
import org.springframework.validation.Errors;
import org.springframework.validation.Validator;
@Service
public class FileValidator implements Validator {
@Override
public boolean supports(Class<?> clazz) {
return false;
}
@Override
public void validate(Object target, Errors errors) {
UploadFIle file = (UploadFIle) target;
if(file.getMpfile().getSize() == 0) {
//mpfile(field)에 대한 errorCode return.
//해당 errorCode가 없으면(파일이 넘어오지 않았다면) default message 전달
errors.rejectValue("mpfile", "fileNPE", "Please select a file");
}
}
}
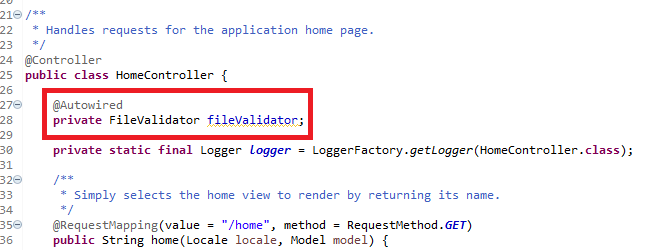
HomeController.java
다시 컨트롤러로 와서 필드를 작성해줍니다.
컨트롤러아래쪽에 해당 코드를 작성해주세요.
@RequestMapping("/upload")
public String fileUpload(HttpServletRequest request, Model model, UploadFile uploadFile, BindingResult result) {
fileValidator.validate(uploadFile, result);
if(result.hasErrors()) {
return "upload";
}
MultipartFile file = uploadFile.getMpfile();
String name = file.getOriginalFilename();
UploadFile fileObj = new UploadFile();
fileObj.setName(name);
fileObj.setDesc(uploadFile.getDesc());
InputStream inputStream = null;
OutputStream outputStream = null;
try {
inputStream = file.getInputStream();
String path = WebUtils.getRealPath(request.getSession().getServletContext(), "/resources/storage");
System.out.println("업로드 실제 경로 : " + path);
File storage = new File(path);
if(!storage.exists()) {
storage.mkdir();
}
File newFile = new File(path+"/"+name);
if(!newFile.exists()) {
newFile.createNewFile();
}
outputStream = new FileOutputStream(newFile);
int read = 0 ;
byte[] b = new byte[(int)file.getSize()];
while((read=inputStream.read(b)) != -1) {
outputStream.write(b,0,read);
}
} catch(IOException e) {
e.printStackTrace();
}
model.addAttribute("fileObj", fileObj);
return "download";
}
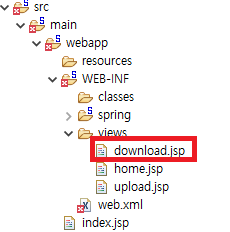
download.jsp를 만들어주세요
download.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
file: ${fileObj.name}<br/>
desc: ${fileObj.desc}<br/>
<form action="download" method="post">
<input type="hidden" name="name" value="${fileObj.name }" />
<input type="submit" value="download" />
</form>
</body>
</html>
다시 컨트롤러에서 다운로드 관련 코드를 작성합니다.
@ResponseBody
@RequestMapping("/download")
public byte[] fileDownload(HttpServletRequest request, HttpServletResponse response, String name) {
byte[] down = null;
try {
String path = WebUtils.getRealPath(request.getSession().getServletContext(), "resources/storage");
File file = new File(path + "/" + name);
down = FileCopyUtils.copyToByteArray(file);
String filename = new String(file.getName().getBytes(), "8859_1");
response.setHeader("Content-Disposition", "attachment; filename=\"" + filename + "\"");
response.setContentLength(down.length);
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
return down;
}
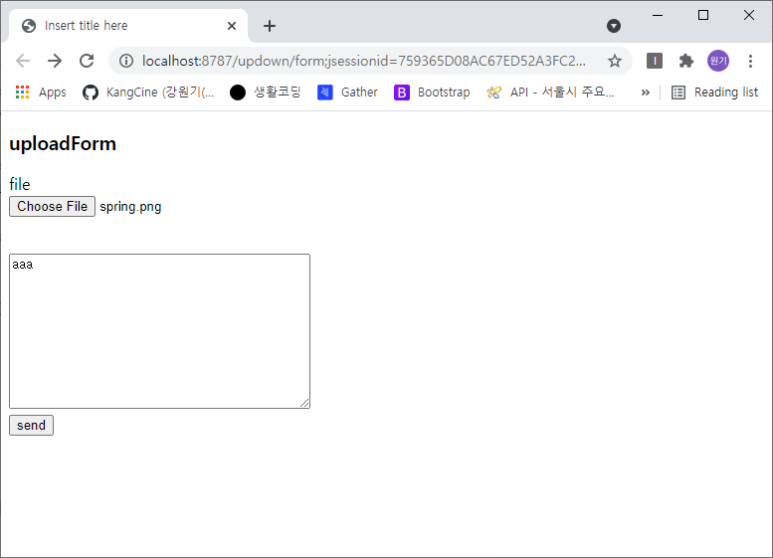
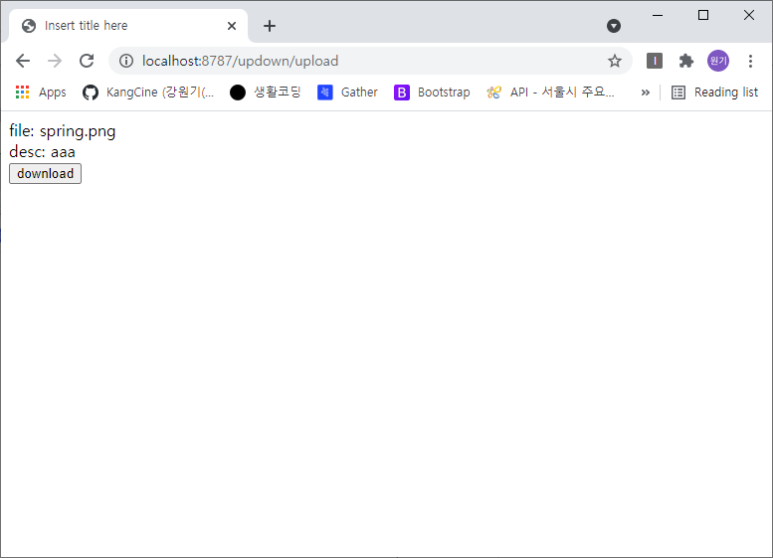
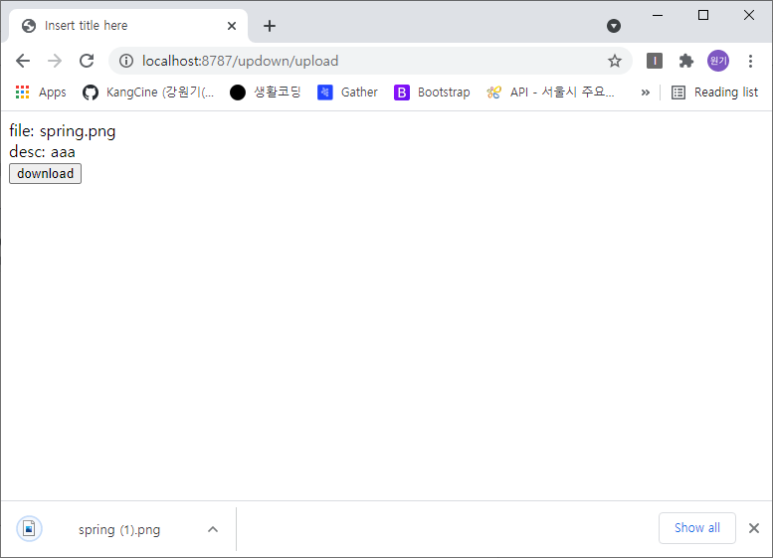
그러면 파일 업로드도 잘 되고 다운로드도 잘 됩니다!
전체코드
'Java 관련 > Spring Legecy' 카테고리의 다른 글
[Spring] Spring JDBC (0) | 2022.04.11 |
---|---|
Spring] update(버전설정 잡기) (0) | 2022.04.10 |
[Spring] security(비밀번호 암호화) (0) | 2022.04.08 |
[Spring] transation(트랜잭션 - 원자성) (0) | 2022.04.07 |
[Spring] Interceptor(인터셉터) (0) | 2022.04.06 |