728x90
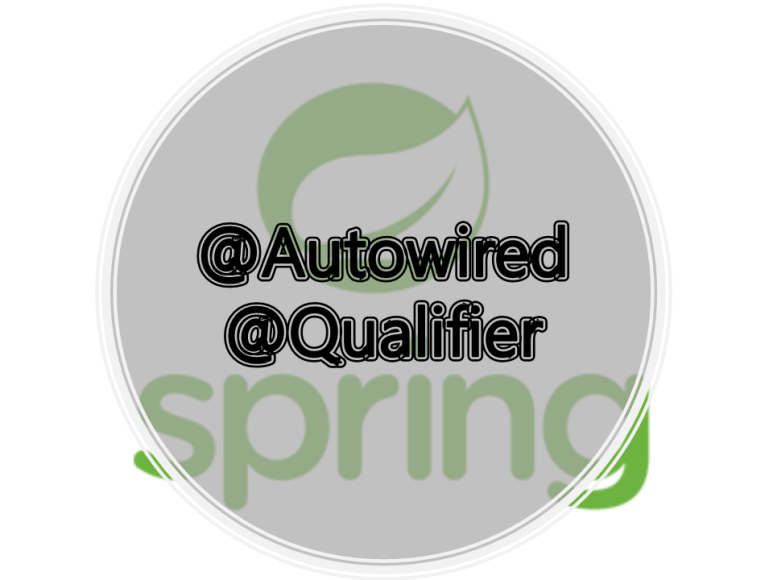
@Autowired
Autowired annotation은 spring에서 의존관계를 자동으로 설정할 때 사용한다.
이 어노테이션은 생성자, 필드, 메서드 세곳에 적용이 가능하며 타입을 이용한 프로퍼티 자동 설정기능을 제공한다.
즉, 해당 타입의 빈 객체가 없거나 2개 이상일 경우 예외를 발생시킨다.
@Qualifier
@Autowired annotation이 타입 기반이기 떄문에 2개 이상의 동일타입 빈 객체가 존재할 시 특정 빈을 사용하도록 선언한다.
@Qualifier("beanName")의 형태로 @AutoWired와 같이 사용하며 메서드에서 두 개 이상의 파라미터를 사용할 경우에는 파라미터 앞에 선언해야 한다.
좀 더 많은 어노테이션에 대한 설명은 Spring_14 스프링 어노테이션에 있습니다!
각 어노테이션에 대한 예시 코드를 볼까요?
패키지 구조
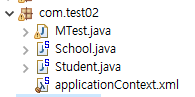
Student.java
package com.test02;
public class Student {
private String name;
private String addr;
private String age;
public Student() {
}
public Student(String name, String addr, String age) {
this.name = name;
this.addr = addr;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddr() {
return addr;
}
public void setAddr(String addr) {
this.addr = addr;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
@Override
public String toString() {
return "Student [name=" + name + ", addr=" + addr + ", age=" + age + "]";
}
}
School.java
package com.test02;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Qualifier;
public class School {
// byType으로 연결할거 있으면 연결하고 아니면 -> byName
@Autowired
@Qualifier("lee")
private Student person;
private int grade;
public School() {
}
public School(Student person, int grade) {
this.person = person;
this.grade = grade;
}
public Student getPerson() {
return person;
}
public void setPerson(Student person) {
this.person = person;
}
public int getGrade() {
return grade;
}
public void setGrade(int grade) {
this.grade = grade;
}
@Override
public String toString() {
return "School [person=" + person + ", grade=" + grade + "]";
}
}
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.3.xsd">
<context:annotation-config />
<bean id="hong" class="com.test02.Student">
<property name="name" value="홍길동" />
<property name="addr" value="서울시 강남구" />
<property name="age" value="100" />
</bean>
<bean id="lee" class="com.test02.Student">
<constructor-arg value="이순신" />
<constructor-arg value="경기도 수원시" />
<constructor-arg value="100" />
</bean>
<bean id="mySchool" class="com.test02.School">
<property name="grade" value="1" />
</bean>
</beans>
MTest.java
package com.test02;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MTest {
public static void main(String[] args) {
ApplicationContext factory = new ClassPathXmlApplicationContext("com/test02/applicationContext.xml");
School mySchool = (School) factory.getBean("mySchool");
System.out.println(mySchool);
}
}
실행결과

applicationContext에 hong객체와 lee객체 두개의 객체가 선언되어있습니다.
하지만 School에서
@Autowired
@Qualifier("lee")
private Student person;
@Autowired가 걸려있습니다. 하지만 찾는 객체가 hong객체와 lee객체 두개이기 때문에 에러가 발생합니다.
따라서 @Qualifier을 통해 어떤 객체랑 연결할건지 선택해줍니다.
hong객체와 연결할 경우 hong객체에 대한 값이 출력이 되겠죠??
그리고 어노테이션을 쓰기 위해서는 xml에서
<context:annotation-config />
가 반드시 필요합니다!! 없으면 에러떠요!
728x90
'Java 관련 > Spring Legecy' 카테고리의 다른 글
[Spring] AOP(cc / ccc) 관점 지향 프로그래밍 (0) | 2022.03.24 |
---|---|
[Spring] Annotation(@Component) (0) | 2022.03.23 |
[Spring] Spring Annotation(스프링 어노테이션) (0) | 2022.03.21 |
[Spring] DI / IoC (Bean 객체_10) - MessageSourceAware (0) | 2022.03.20 |
[Spring] DI / IoC (Bean 객체_10) - autowire (0) | 2022.03.19 |