728x90
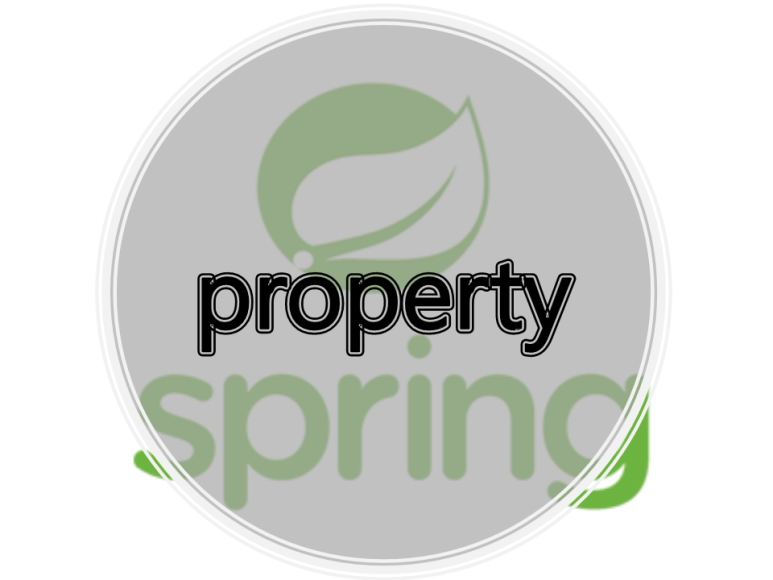
지난 포스팅에서는 생성자 주입을 통한 Bean객체 생성을 했지만 생성자가 아닌 setter에 값을 넣을수도 있습니다.
패키지 구조
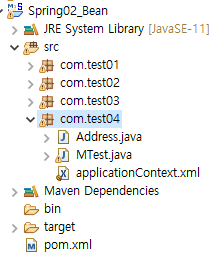
Address.java
package com.test04;
public class Address {
private String name;
private String addr;
private String phone;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddr() {
return addr;
}
public void setAddr(String addr) {
this.addr = addr;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String toString() {
return "이름 : " + name + " \t 주소 : " + addr + "\t 전화번호 : " + phone;
}
}
applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="lee" class="com.test04.Address">
<property name="name" value="이순신"></property>
<property name="addr" value="서울시 강남구"></property>
<property name="phone" value="010-1111-1111"></property>
</bean>
<bean id="hong" class="com.test04.Address">
<property name="name" value="홍길동"></property>
<property name="addr" value="경기도 수원시"></property>
<property name="phone" value="010-2222-2222"></property>
</bean>
</beans>
MTest.java
package com.test04;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MTest {
public static void main(String[] args) {
ApplicationContext factory = new ClassPathXmlApplicationContext("com/test04/applicationContext.xml");
Address lee = (Address) factory.getBean("lee");
Address hong = (Address) factory.getBean("hong");
System.out.println(lee);
System.out.println(hong);
}
}
실행결과

해당 코드에서는 <constructor-arg>태그 대신에 <property>태그를 사용하였습니다.
constructor-arg는 생성자의 파라미터에 아규먼트값을 주는것이라면 property는 setter에 값을 주입하는 방법입니다.
<bean id="lee" class="com.test04.Address">
<property name="name" value="이순신"></property>
<property name="addr" value="서울시 강남구"></property>
<property name="phone" value="010-1111-1111"></property>
</bean>
setter의 이름이 name인 것을 찾아 "이순신"이라는 value(값)을 주입합니다.
여기서 name이라는 변수는 setName이라는 세터의 이름과 자동으로 매칭이 됩니다(오타주의)
setAddr에는 "서울시 강남구"를 주입하겠죠?
setPhone()메소드에는 "010-1111-1111"의 값이 들어갑니다.
728x90
'Java 관련 > Spring Legecy' 카테고리의 다른 글
[Spring] DI / IoC (Bean 객체 생성_05) - construct-arg와 property 동시 사용도 될까? (0) | 2022.03.14 |
---|---|
[Spring] DI / IoC (Bean 객체 생성_04) - Singleton (0) | 2022.03.13 |
[Spring] DI / IoC (Bean 객체 생성_02) - 생성자 주입 (0) | 2022.03.11 |
[Spring] DI / IoC (Bean 객체 생성_01) (0) | 2022.03.10 |
[Spring] 기본세팅(Maven / pom.xml) (0) | 2022.03.09 |