728x90

패키지 구조
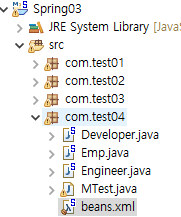
Emp.java
package com.test04;
public class Emp {
private String name;
private int salary;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getSalary() {
return salary;
}
public void setSalary(int salary) {
this.salary = salary;
}
@Override
public String toString() {
return "이름 : " + name + "\t 월급 : " + salary;
}
}
Developer.java
package com.test04;
public class Developer {
private Emp emp;
private String dept;
public Developer() {
}
public Developer(Emp emp, String dept) {
this.emp = emp;
this.dept = dept;
}
@Override
public String toString() {
return emp + " \t 부서 : " + dept;
}
}
Engineer.java
package com.test04;
public class Engineer {
private Emp emp;
private String dept;
public Engineer() {
}
public Engineer(Emp emp, String dept) {
this.emp = emp;
this.dept = dept;
}
@Override
public String toString() {
return emp + " \t 부서 : " + dept;
}
}
beans.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="lee" class="com.test04.Emp">
<property name="name" value="이순신" />
<property name="salary" value="3000000" />
</bean>
<bean id="hong" class="com.test04.Emp">
<property name="name" value="홍길동" />
<property name="salary" value="2500000" />
</bean>
<bean id="lee-ss" class="com.test04.Developer">
<constructor-arg name="emp" ref="lee" />
<constructor-arg name="dept" value="개발" />
</bean>
<bean id="hong-gd" class="com.test04.Engineer">
<constructor-arg index="0" ref="hong" />
<constructor-arg index="1" value="기술" />
</bean>
</beans>
MTest.java
package com.test04;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MTest {
public static void main(String[] args) {
ApplicationContext factory = new ClassPathXmlApplicationContext("com/test04/beans.xml");
Developer lee = factory.getBean("lee-ss", Developer.class);
Engineer hong = (Engineer) factory.getBean("hong-gd");
System.out.println(lee);
System.out.println(hong);
}
}
실행결과

각 클래스의 생성자와 세터의 여부에 따라 객체를 반들때 주입방식이 정말 다양해 질수 있습니다.
<bean id="lee" class="com.test04.Emp">
<property name="name" value="이순신" />
<property name="salary" value="3000000" />
</bean>
<bean id="hong" class="com.test04.Emp">
<property name="name" value="홍길동" />
<property name="salary" value="2500000" />
</bean>
해당 코드에서는 Emp클래스를 이용하여 lee객체와 hong객체를 만들었는데요.
이런 객체를 자식 클래스를 이용한 객체에서 참조 할 수 있습니다. ref속성으로 말이죠!
<bean id="lee-ss" class="com.test04.Developer">
<constructor-arg name="emp" ref="lee" />
<constructor-arg name="dept" value="개발" />
</bean>
<bean id="hong-gd" class="com.test04.Engineer">
<constructor-arg index="0" ref="hong" />
<constructor-arg index="1" value="기술" />
</bean>
Engineer클래스와 Developer클래스 모두 파라미터가 2개짜리인 생성자에 모면 Emp타입의 emp변수를 파라미터로 받고있습니다.
lee-ss객체와 hong-gd객체에서는 Emp타입의 emp변수에 lee객체와 hong객체를 참조하여 아규먼트로 값을 주입하였습니다!
MTest에서도 객체를 사용할때 불러오는 방법 두가지 모두 같이 사용 할 수 있습니다.
Developer lee = factory.getBean("lee-ss", Developer.class);
Engineer hong = (Engineer) factory.getBean("hong-gd");
728x90
'Java 관련 > Spring Legecy' 카테고리의 다른 글
[Spring] DI / IoC (Bean 객체 생성_08) - <bean>태그 속성 (0) | 2022.03.17 |
---|---|
[Spring] DI / IoC (Bean 객체 생성_07) - schema/c(c:), schema/p(p:) (0) | 2022.03.16 |
[Spring] DI / IoC (Bean 객체 생성_05) - construct-arg와 property 동시 사용도 될까? (0) | 2022.03.14 |
[Spring] DI / IoC (Bean 객체 생성_04) - Singleton (0) | 2022.03.13 |
[Spring] DI / IoC (Bean 객체 생성_03) - setter 주입 (0) | 2022.03.12 |