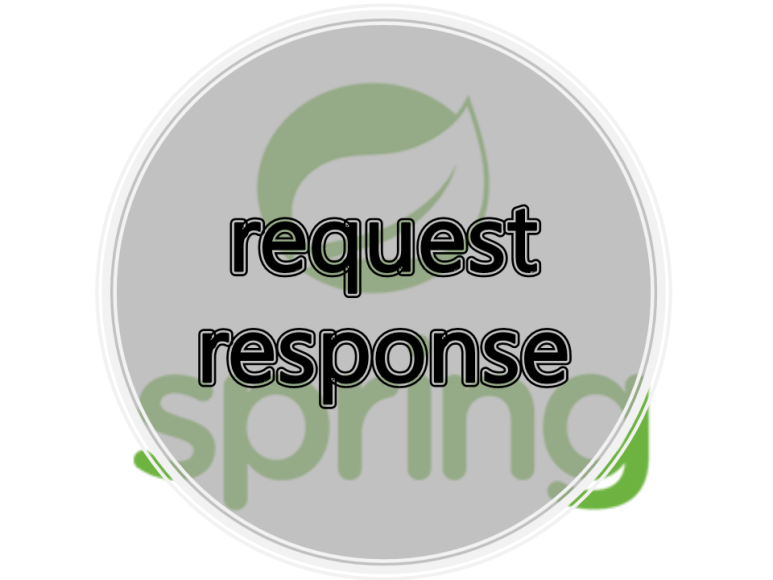
Spring Legacy Project를 만들어주세요!
web.xml에 encodingFilter를 설정해주시고 순서대로 따라가 보겠습니다.
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="2.5" xmlns="http://JAVA.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee https://java.sun.com/xml/ns/javaee/web-app_2_5.xsd">
<!-- The definition of the Root Spring Container shared by all Servlets and Filters -->
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/root-context.xml</param-value>
</context-param>
<!-- Creates the Spring Container shared by all Servlets and Filters -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>
<!-- Processes application requests -->
<servlet>
<servlet-name>appServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/spring/appServlet/servlet-context.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>appServlet</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
<!-- encoding filter -->
<filter>
<filter-name>encoding</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>encodingFilter</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>coding</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
</web-app>
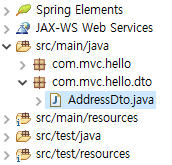
그리고 com.mvc.hello.dto패키지를 만들고 그 안에 AddressDto파일을 만들어주세요!
AddressDto.java
package com.mvc.hello.dto;
public class AddressDto {
private String name;
private String addr;
private String phone;
public AddressDto() {
}
public AddressDto(String name, String addr, String phone) {
this.name = name;
this.addr = addr;
this.phone = phone;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddr() {
return addr;
}
public void setAddr(String addr) {
this.addr = addr;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
}
index.jsp도 만들어 주세요!
index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<a href="command.do?name=Spring&addr=서울&phone=010-1234-5678">get</a>
<br/>
<form action="command.do" method="post">
이름:<input type="text" name="name"/><br>
주소:<input type="text" name="addr"/><br>
번호:<input type="text" name="phone"/><br>
<input type="submit" value="전송"/>
</form>
<a href="void.do">void</a>
</body>
</html>
HomeController에 메소드를 추가해주세요!
package com.hello.mvc2;
import java.text.DateFormat;
import java.util.Date;
import java.util.Locale;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import com.hello.mvc2.dto.AddressDto;
@Controller
public class HomeController {
private static final Logger logger = LoggerFactory.getLogger(HomeController.class);
@RequestMapping(value = "/", method = RequestMethod.GET)
public String home(Locale locale, Model model) {
logger.info("Welcome home! The client locale is {}.", locale);
Date date = new Date();
DateFormat dateFormat = DateFormat.getDateTimeInstance(DateFormat.LONG, DateFormat.LONG, locale);
String formattedDate = dateFormat.format(date);
model.addAttribute("serverTime", formattedDate );
return "home";
}
@RequestMapping(value="/command.do", method=RequestMethod.GET)
public String getCommand(Model model, @RequestParam("name") String name, String addr, String phone) {
model.addAttribute("dto", new AddressDto(name, addr, phone));
return "get";
}
@RequestMapping(value="/command.do", method=RequestMethod.POST)
public String postCommand(Model model, @ModelAttribute AddressDto dto) {
model.addAttribute("dto", dto);
return "post";
}
@RequestMapping("/void.do")
public void voidPage(Model model) {
model.addAttribute("message", "viewname 안써도 넘어갑니다.");
}
}
그리고 views에 get.jsp파일과 post.jsp파일 / void.jsp 만들어주세요!!
(get.jsp와 post.jsp는 내용 똑같이 만들어주세요!)
get.jsp / post.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<table>
<tr>
<th>이름</th>
<td>${dto.name }</td>
</tr>
<tr>
<th>주소</th>
<td>${dto.addr }</td>
</tr>
<tr>
<th>전화번호</th>
<td>${dto.phone }</td>
</tr>
</table>
</body>
</html>
void.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<h1>${message }</h1>
</body>
</html>
그럼 이제 실행시켜볼까요??
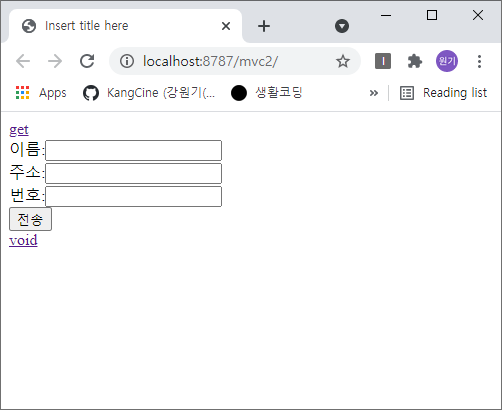
가장 먼저 실행시키면 해당 화면이 나옵니다.
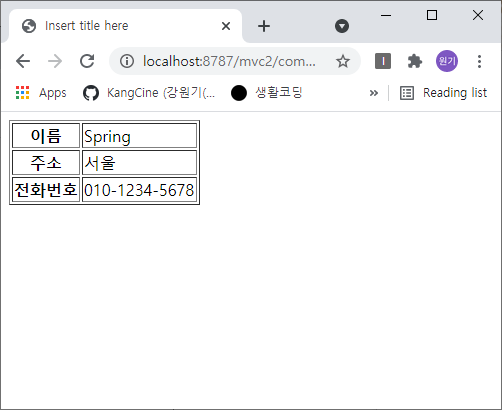
get버튼 클릭시
get버튼 클릭시에는 quseryString으로 값을 넘겨줍니다.
method속성의 default값인 get방식으로 controller에 넘겨주게 됩니다.

Controller에서 넘겨받을때 method=RequestMethod.GET으로 인해 get방식으로만 받게 되며 메서드의 파라미더의 형태가 모두 다르지만 같은 역할을 합니다.
index에서 quesryString으로 넘겨 받은 값을 받아주는 명령이며 빨간부분, 연두색부분, 파란색 부분은 형태는 다르지만 모두 같은 역할을 합니다.
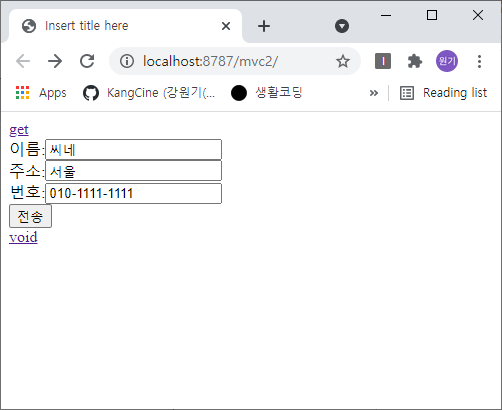
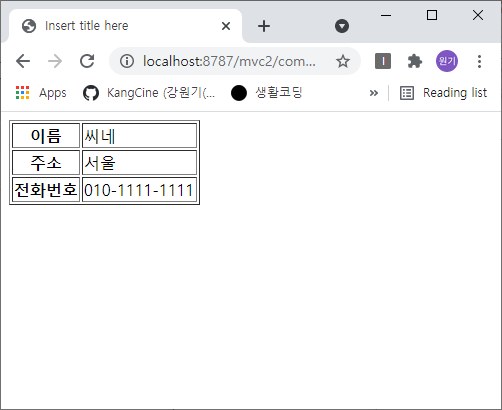
text박스에 value작성후 전송버튼을 눌렀을때
form태그를 통해 전달 받은 값들이 잘 전달 되었습니다.
하지만 form 태그의 method="post"가 없다면 default인 get방식으로 넘어가게 되어 post방식으로 받아주는 메서드가 없다면 405에러가 발생합니다.
그래서 post방식으로 전달받는 똑같은 형태의 메서드를 만들었으며 method=RequestMethod.GET이나
method=RequestMethod.POST가 없다면 get방식 post방식 모두 받아주는 형태가 됩니다.
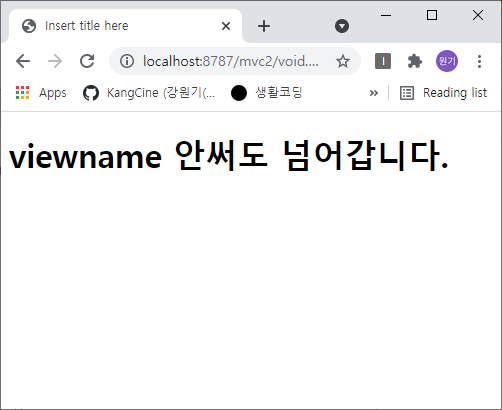
void 눌렀을때
void의 링크는 void.do로 요청했습니다.
@RequestMapping("/void.do")
public void voidPage(Model model) {
model.addAttribute("message", "viewname 안써도 넘어갑니다.");
}
컨트롤러에서 해당 메서드에는 return이 없는데 만약 return "";이 없다면 Mapping되어있는 void을 찾게 됩니다.
void.jsp로 가라는 명령이 없는데도 실행하면 void.jsp로 가는 모습을 볼 수 있습니다!
'Java 관련 > Spring Legecy' 카테고리의 다른 글
[Spring] filter(javax.servlet.Filter) (0) | 2022.04.03 |
---|---|
[Spring] 스프링으로 게시판만들기 (0) | 2022.04.02 |
[Spring] encodingFilter로 한글 설정하기 (0) | 2022.03.31 |
[Spring] Spring Legacy Project (0) | 2022.03.30 |
[Spring] Spring MVC & TODO (@RequestMapping @RequestParam @ModelAttribute @SessionAttribute) (0) | 2022.03.29 |