728x90
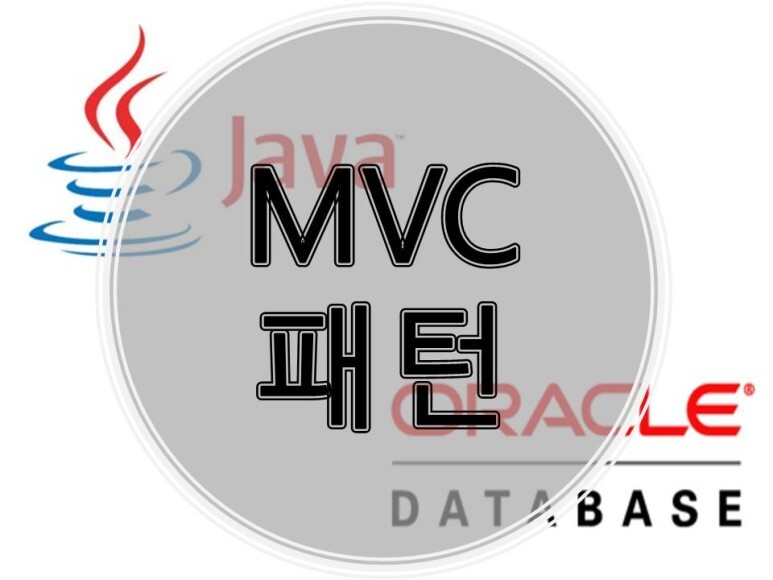
MVC패턴은 Model View Controller의 앞글자를 따서 지어진 말입니다.
Model : 연산 처리, DB연결 등 백그라운드에서 동작하는 로직을 처리합니다.
View : 사용자가 보게 될 결과 화면을 출력합니다.
Controller : 사용자의 입력처리와 흐름 제어를 담당합니다.
이후 JSP에서 많이 사용될 예정인 MVC패턴을 자바로 구현해보겠습니다.
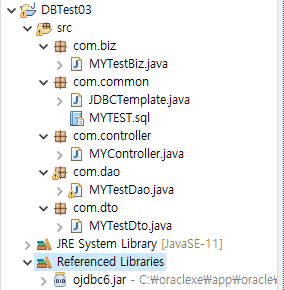
우선 각 파일의 구성은 다음과 같습니다.
//MYTEST.sql
DROP TABLE MYTEST;
CREATE TABLE MYTEST(
MNO NUMBER,
MNAME VARCHAR2(20),
NICKNAME VARCHAR2(20)
);
SELECT MNO, MNAME, NICKNAME
FROM MYTEST;
INSERT INTO MYTEST
VALUES(1, '관리자', '관리자입니다.');
데이터베이스입니다. 간략한 구현을 위해 다음과 같이 만들었습니다.
// JDBCTemplate.java
package com.common;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
// DB연결, 연결해제, 저장(commit), 취소(rollback)
// method를 static으로 만들자.
public class JDBCTemplate {
public static Connection getConnection() {
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
String url = "jdbc:oracle:thin:@localhost:1521:xe";
String user = "kh";
String password = "kh";
Connection con = null;
try {
con = DriverManager.getConnection(url, user, password);
con.setAutoCommit(false);
} catch (SQLException e) {
e.printStackTrace();
}
return con;
}
public static void close(Connection con) {
try {
con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void close(Statement stmt) {
try {
stmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void close(ResultSet rs) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void commit(Connection con) {
try {
con.commit();
} catch (SQLException e) {
e.printStackTrace();
}
}
public static void rollback(Connection con) {
try {
con.rollback();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
JDBCTemplate의 경우 JDBC의 1단계, 2단계, 5단계인 Driver연결, 계정연결, DB종료가 담겨있습니다.
// MYTestDto.java
package com.dto;
// Data Transfer Object : 값 전달 객체
// Value Object : 값 객체
// db table의 row 한개를 저장할 수 있다.
public class MYTestDto {
private int mno;
private String mname;
private String nickname;
// 기본생성자, 파라미터 3개 생성자
public MYTestDto() {
}
public MYTestDto(int mno, String mname, String nickname){
this.mno = mno;
this.mname = mname;
this.nickname = nickname;
}
// getter & setter
public int getMno() {
return this.mno;
}
public void setMno(int mno) {
this.mno = mno;
}
public String getMname() {
return this.mname;
}
public void setMname(String mname) {
this.mname = mname;
}
public String getNickname() {
return this.nickname;
}
public void setNickname(String nickname) {
this.nickname = nickname;
}
}
// MYTestBiz.java
package com.biz;
import java.util.List;
import com.dao.MYTestDao;
import com.dto.MYTestDto;
// Biz : Business Logic -> 연산/처리
public class MYTestBiz {
private MYTestDao dao = new MYTestDao();
public List<MYTestDto> selectList(){
return dao.selectList();
}
public MYTestDto selectOne(int mno) {
return dao.selectOne(mno);
}
public int insert(MYTestDto dto) {
return dao.insert(dto);
}
public int update(MYTestDto dto) {
return dao.update(dto);
}
public int delete(int mno) {
return dao.delete(mno);
}
}
// MYTestDao.java
package com.dao;
import static com.common.JDBCTemplate.*;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.List;
import java.util.Scanner;
import com.dto.MYTestDto;
// Data Access Object : DB와 접근하는 객체.
// import static : class.method 하고 호출 할 때, class 생략하게 만들어줌
public class MYTestDao {
//전체출력
public List<MYTestDto> selectList(){
// 1. 2.
//Connection con = JDBCTemplate.getCommection(); // -> import static com.common.JDBCTemplate.*;덕분에 간략하게 씀
Connection con = getConnection();
String sql = " SELECT MNO, MNAME, NICKNAME "
+ " FROM MYTEST ";
Statement stmt = null;
ResultSet rs = null;
List<MYTestDto> list = new ArrayList<MYTestDto>();
try {
// 3.
stmt = con.createStatement();
// 4.
rs = stmt.executeQuery(sql);
while(rs.next()) {
MYTestDto temp = new MYTestDto();
temp.setMno(rs.getInt("MNO"));
temp.setMname(rs.getString("MNAME"));
temp.setNickname(rs.getString("NICKNAME"));
list.add(temp);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
close(rs);
close(stmt);
close(con);
}
return list;
}
//선택출력
public MYTestDto selectOne(int mno){
// 1. 2.
Connection con = getConnection();
String sql = " SELECT MNO, MNAME, NICKNAME "
+ " FROM MYTEST "
+ " WHERE MNO = ? ";
PreparedStatement pstm = null;
ResultSet rs = null;
MYTestDto dto = new MYTestDto();
try {
// 3.
pstm = con.prepareStatement(sql);
pstm.setInt(1, mno);
// 4.
rs = pstm.executeQuery();
while(rs.next()) {
dto.setMno(rs.getInt("MNO"));
dto.setMname(rs.getString("MNAME"));
dto.setNickname(rs.getString("NICKNAME"));
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
close(rs);
close(pstm);
close(con);
}
return dto;
}
//추가
public int insert(MYTestDto dto) {
Connection con = getConnection();
String sql = " INSERT INTO MYTEST "
+ " VALUES(?, ?, ?) ";
PreparedStatement pstm = null;
int res = 0;
try {
pstm = con.prepareStatement(sql);
pstm.setInt(1, dto.getMno());
pstm.setString(2, dto.getMname());
pstm.setString(3, dto.getNickname());
//4.
res = pstm.executeUpdate();
if( res > 0) {
commit(con);
}
} catch (SQLException e) {
e.printStackTrace();
} finally {
//5.
close(pstm);
close(con);
}
return res;
}
//수정
public int update(MYTestDto dto) {
Connection con = getConnection();
String sql = " UPDATE MYTEST "
+ " SET MNAME = ?, NICKNAME = ? "
+ " WHERE MNO = ? ";
PreparedStatement pstm = null;
int res = 0;
try {
pstm = con.prepareStatement(sql);
pstm.setString(1, dto.getMname());
pstm.setString(2, dto.getNickname());
pstm.setInt(3, dto.getMno());
res = pstm.executeUpdate();
if(res > 0)
commit(con);
}catch (SQLException e) {
e.printStackTrace();
} finally {
//5.
close(pstm);
close(con);
}
return res;
}
//삭제
public int delete(int mno) {
Connection con = getConnection();
PreparedStatement pstm = null;
String sql = " DELETE FROM MYTEST "
+ " WHERE MNO = ? ";
int res = 0;
try {
pstm = con.prepareStatement(sql);
pstm.setInt(1, mno);
res = pstm.executeUpdate();
if(res > 0) {
commit(con);
}
}catch (SQLException e) {
e.printStackTrace();
} finally {
//5.
close(pstm);
close(con);
}
return res;
}
}
// MYController.java
package com.controller;
import java.util.List;
import java.util.Scanner;
import com.biz.MYTestBiz;
import com.dto.MYTestDto;
public class MYController {
private static Scanner sc = new Scanner(System.in);
//view
public static int getMenu() {
StringBuffer sb = new StringBuffer();
sb.append("1.전체출력\n")
.append("2.선택출력\n")
.append("3.추 가\n")
.append("4.수 정\n")
.append("5.삭 제\n")
.append("6.종 료\n")
.append("input select : ");
System.out.println(sb);
int select = sc.nextInt();
return select;
}
public static void main(String[] args) {
int select = 0;
MYTestBiz biz = new MYTestBiz();
do {
select = getMenu();
switch(select) {
case 1:
//전체출력
List<MYTestDto> list = biz.selectList();
for(MYTestDto dto : list) {
System.out.printf("%3d %10s %10s\n", dto.getMno(), dto.getMname(), dto.getNickname());
}
break;
case 2:
// 선택출력
System.out.println("출력할 부서번호 : ");
int selectOneNo = sc.nextInt();
MYTestDto selectOneDto = biz.selectOne(selectOneNo);
System.out.printf("%3d %10s %10s\n", selectOneDto.getMno(), selectOneDto.getMname(), selectOneDto.getNickname());
break;
case 3:
// 추가
System.out.println("추가할 번호 : ");
int insertNo = sc.nextInt();
System.out.println("추가할 이름 : ");
String insertName = sc.next();
System.out.println("추가할 별명 : ");
String insertNickname = sc.next();
MYTestDto insertDto = new MYTestDto();
insertDto.setMno(insertNo);
insertDto.setMname(insertName);
insertDto.setNickname(insertNickname);
int insertRes = biz.insert(insertDto);
if(insertRes > 0) {
System.out.println("추가 성공");
} else {
System.out.println("추가 실패");
}
break;
case 4:
//수정
System.out.println("수정할 번호 : ");
int updateNo = sc.nextInt();
System.out.println("수정할 이름 : ");
String updateName = sc.next();
System.out.println("수정할 별명");
String updateNickname = sc.next();
MYTestDto updateDto = new MYTestDto(updateNo, updateName, updateNickname);
int updateRes = biz.update(updateDto);
if(updateRes > 0) {
System.out.println("수정 성공");
}else {
System.out.println("수정 실패");
}
break;
case 5:
//삭제
System.out.println("삭제할 번호 : ");
int deleteNO = sc.nextInt();
int deleteRes = biz.delete(deleteNO);
if(deleteRes > 0 ) {
System.out.println("삭제 성공");
}else {
System.out.println("삭제 실패");
}
break;
case 6:
System.out.println("프로그램이 종료되었습니다...");
}
}while(select != 6);
}
}
728x90
'Java 관련 > JDBC' 카테고리의 다른 글
[JDBC] delete(데이터 삭제) (0) | 2022.03.05 |
---|---|
[JDBC] update(데이터 수정) (0) | 2022.03.04 |
[JDBC] selectOne(선택 출력하기) (0) | 2022.03.02 |
[JDBC] Statement & PreparedStatement (0) | 2022.03.01 |
[JDBC] JDBC(Java DataBase Connectivity)연결순서 (0) | 2022.02.28 |