728x90
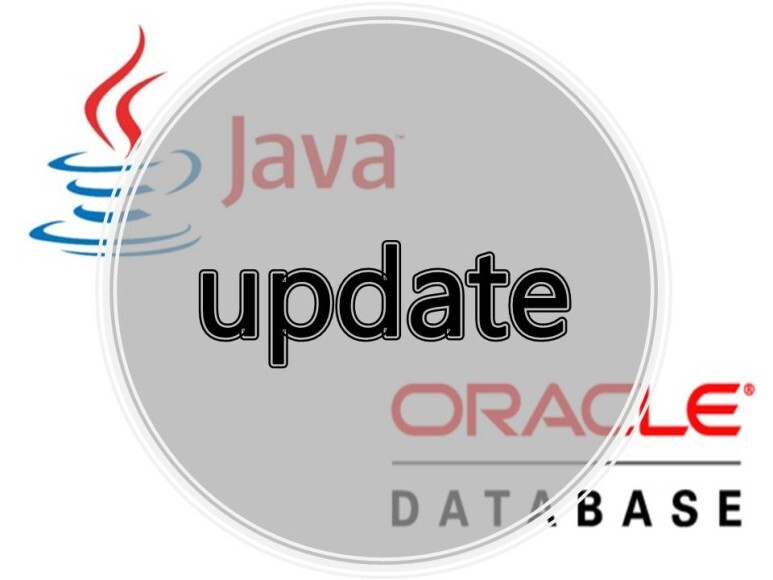
1. Statement 방식
package com.test01;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.Scanner;
public class MTest05 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("수정할 부서번호 : ");
int deptno = sc.nextInt();
System.out.println("수정할 부서이름 : ");
String dname = sc.next();
System.out.println("수정할 부서지역 : ");
String loc = sc.next();
//1. 드라이버연결
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
//2. 계정연결
String url = "jdbc:oracle:thin:@localhost:1521:xe";
String user = "kh";
String password = "kh";
Connection con = null;
try {
con = DriverManager.getConnection(url, user, password);
// con.setAutoCommit(false);
} catch (SQLException e) {
e.printStackTrace();
}
String sql = " UPDATE DEPT "
+ " SET DNAME = '" + dname + "', LOC = '" + loc
+ "' WHERE DEPTNO = " + deptno;
Statement stmt = null;
try {
//3. Query 준비
stmt = con.createStatement();
//4. Query 실행 및 리턴
int res = stmt.executeUpdate(sql);
if(res > 0) {
System.out.println("입력 성공");
}else {
System.out.println("입력 실패");
}
}catch (Exception e) {
e.printStackTrace();
}finally {
//5. DB종료
try {
stmt.close();
con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
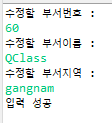
2. PreparedStatement 방식
package com.test01;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Scanner;
public class MTest05 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("수정할 부서번호 : ");
int deptno = sc.nextInt();
System.out.println("수정할 부서이름 : ");
String dname = sc.next();
System.out.println("수정할 부서지역 : ");
String loc = sc.next();
//1. 드라이버연결
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
//2. 계정연결
String url = "jdbc:oracle:thin:@localhost:1521:xe";
String user = "kh";
String password = "kh";
Connection con = null;
try {
con = DriverManager.getConnection(url, user, password);
// con.setAutoCommit(false);
} catch (SQLException e) {
e.printStackTrace();
}
String sql = " UPDATE DEPT "
+ " SET DNAME = ?, LOC = ? "
+ " WHERE DEPTNO = ? ";
PreparedStatement pstm = null;
try {
//3. Query 준비
pstm = con.prepareStatement(sql);
pstm.setString(1, dname);
pstm.setString(2, loc);
pstm.setInt(3, deptno);
//4. Query 실행
int res = pstm.executeUpdate();
if(res > 0) {
System.out.println("수정 성공");
} else {
System.out.println("수정 실패");
}
} catch (SQLException e) {
e.printStackTrace();
}finally {
//5. DB종료
try {
pstm.close();
con.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
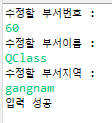
728x90
'Java 관련 > JDBC' 카테고리의 다른 글
[JDBC] MVC 패턴(Model View Controller) (0) | 2022.03.06 |
---|---|
[JDBC] delete(데이터 삭제) (0) | 2022.03.05 |
[JDBC] selectOne(선택 출력하기) (0) | 2022.03.02 |
[JDBC] Statement & PreparedStatement (0) | 2022.03.01 |
[JDBC] JDBC(Java DataBase Connectivity)연결순서 (0) | 2022.02.28 |