728x90

Spring_18보다 살짝 심화된 내용입니다.
이전 포스팅을 먼저 보고 오시는 것을 권장드립니다.
패키지 구조

Student.java
package com.test03;
public interface Student {
void classWork();
}
Woman.java
package com.test03;
public class Woman implements Student {
@Override
public void classWork() {
System.out.println("컴퓨터를 켜서 주식본다.");
}
}
Man.java
package com.test03;
public class Man implements Student {
@Override
public void classWork() {
System.out.println("컴퓨터를 켜서 뉴스본다.");
}
}
MyAspect.java
package com.test03;
import org.aspectj.lang.JoinPoint;
public class MyAspect {
public void before(JoinPoint join) {
System.out.println("출석한다.");
System.out.println(join.getTarget().getClass());
System.out.println(join.getSignature().getName());
}
public void after() {
System.out.println("집에간다.");
}
}
applicationContext.xml (Namespaces에서 aop 체크해주세요)
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.3.xsd">
<bean id="woman" class="com.test03.Woman" />
<bean id="man" class="com.test03.Man" />
<bean id="myAspect" class="com.test03.MyAspect" />
<aop:config>
<aop:aspect ref="myAspect">
<!-- 첫번째 *->리턴타입 (..)은 파라미터의 갯수가 몇개여도 상관없다 -->
<!-- execution(public * com.test03.Woman.classWork(..)) -->
<!-- Woman.classWork가 호출될때만 before붙여주세요 하는 명령 -->
<aop:before method="before" pointcut="execution(public void com.test03.Woman.classWork())" />
<aop:after method="after" pointcut="execution(public void com.test03.Woman.classWork())" />
</aop:aspect>
</aop:config>
</beans>
MTest.java
package com.test03;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MTest {
public static void main(String[] args) {
ApplicationContext factory = new ClassPathXmlApplicationContext("com/test03/applicationContext.xml");
Student w = factory.getBean("woman", Student.class);
Student m = (Student) factory.getBean("man");
System.out.println("여학생 입장");
w.classWork();
System.out.println("---------");
System.out.println("남학생입장");
m.classWork();
}
}
실행결과

이번 코드에서도 이전 코드와 매우 비슷합니다.
Man과 Woman은 주 관심사항(cc)이고 MyAspect는 공통관심사항(ccc)입니다.
<aop:config>
<aop:aspect ref="myAspect">
<aop:before method="before" pointcut="execution(public void com.test03.Woman.classWork())" />
<aop:after method="after" pointcut="execution(public void com.test03.Woman.classWork())" />
</aop:aspect>
</aop:config>
xml의 다음 코드에서 myAspect객체를 연결했는데 누구한테 연결을 할지에 관한 코드입니다.
execution(public void com.test03.Woman.classWork())의 경우
execution(public * *(..))이라고 할 경우 모든 리턴타입의 모든 메서드에 연결하게됩니다.
하지만 위의 코드에서는 before, after 모두 Woman클래스의 classWork()메서드와 연결이 됩니다.
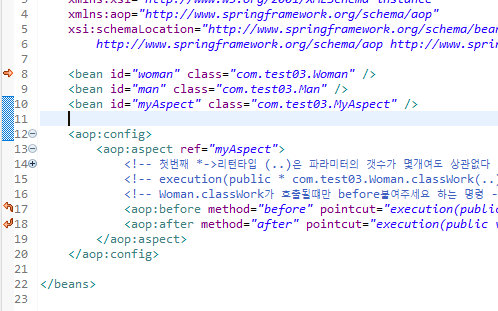
이클리스에서 보면 좌츶에 화살표로 표시가 되어있는데 연결이 되었다는 표시입니다.

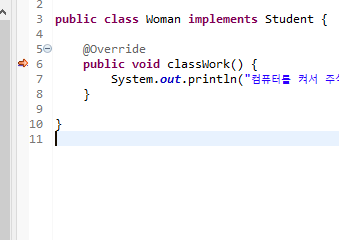
MyAspect클래스와 Woman클래스에서 화살표가 있네요!
Woman에만 연결이 되어있어서 Man의 m을 호출할때는 proxy 객체가 만들어지지 않게됩니다
728x90
'Java 관련 > Spring Legecy' 카테고리의 다른 글
[Spring] AOP(@Component) (0) | 2022.03.28 |
---|---|
[Spring] AOP(before, after, after-returning, after-throwing, around) (0) | 2022.03.27 |
[Spring] AOP(joinpoin, pointcut, advice, aspect, weaving) (0) | 2022.03.25 |
[Spring] AOP(cc / ccc) 관점 지향 프로그래밍 (0) | 2022.03.24 |
[Spring] Annotation(@Component) (0) | 2022.03.23 |